Learn how to make a simple animated background color loop with pure CSS by using keyframes and various CSS animation properties.
In this example, we’re targeting the HTML <body>
element directly with CSS, but you can apply the following code example to any HTML element, class, or id.
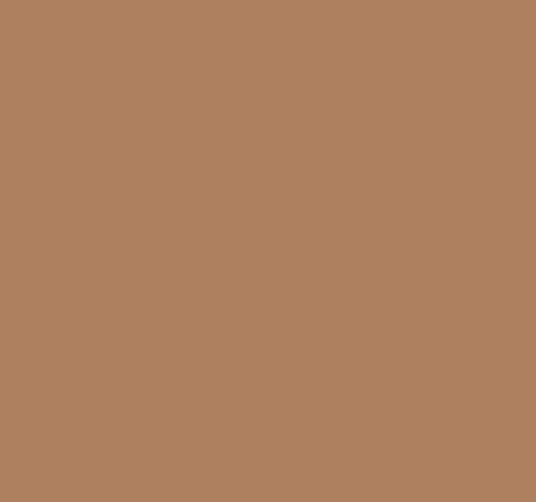
The Code
You can use this demo as a reference.
Note: this code won’t work in IE9.
Planning your animation
Even if you don’t know exactly how your result should end up it’s always practical to have an idea about the direction you’re going. Let’s establish a couple of things about the looping animation before we start coding:
- How many background colors do we want to use?
- How long should total animation duration last?
- What type of animation type should we use?
Since the purpose of this tutorial is to keep things simple, let’s use:
- 5 different background colors
- A duration of 10 seconds (each color gets displayed 2 seconds)
- A linear animation curve (the animation has the same speed from start to end)
I used Coolers.co to quickly generate a harmonious color palette for this example:
These are the hex colors our upcoming background animation will loop through:
- sunset orange:
#EE6055
- medium aquamarine:
#60D394
- pale green:
#AAF683
- mellow yellow:
#FFD97D
- vivid tangerine:
#FF9B85
You don’t need to memorize all those names (they are taken directly from Coolers), I just added them for good measure.
Okay, so we now have our 5 colors, that we’re going to throw into a looping animation that displays each color 2 seconds.
Next up we’ll create the animation based on our plan.
Creating the looping CSS animation
In CSS, animations keyframes work in percentages from 0%
to 100%
. Add the following CSS keyframes to your stylesheet:
/* Standard syntax */
@keyframes backgroundColorPalette {
0% {
background: #ee6055;
}
25% {
background: #60d394;
}
50% {
background: #aaf683;
}
75% {
background: #ffd97d;
}
100% {
background: #ff9b85;
}
}
Now we have a keyframes property called backgroundColorPalette
with 5 color intervals, which are evenly divided over from 0%
to 100%
of the animation.
Now it’s time to create the body element’s CSS rule-set, so we can take our keyframes color palette and put it to use.
Add the following CSS animation properties inside your body selector rule-set, and look what happens in your browser window:
body {
animation-name: backgroundColorPalette;
animation-duration: 10s;
animation-iteration-count: infinite;
animation-direction: alternate;
}
If you did everything right, you should now have continuously running background color animation that smoothly transitions from color to color with 2 second intervals.
The Code
You can use this demo as a reference.How the CSS works
- First, we add the
animation-name
property and give it a value of thebackgroundColorPalette
— now the background color keyframes we created earlier are assigned to the body element. - We use the
animation-duration:
property and give it a value of 10s — now our animation’s total duration is 10 seconds. You can also use milliseconds10000
. - We use the
animation-iteration-count
property and give it a value ofinfinite
. This is what makes the animation loop continuously. In CSS, the default is1
animation cycle. - We use the
animation-direction
property and give it a value ofalternate
. This makes the animation play from beginning to end, and from the end to the beginning. We use this property value to avoid an ugly jump which happens if you use thenormal
animation direction value.
Good to know
By default, the CSS animation speed curve type is set to linear. This means that we don’t have to declare the property in our CSS rule-sets when we want to use it. That’s why the animation speed curve in our example earlier runs linearly.
However, you might still want to add animation-timing-function: linear;
to your CSS rule-set to make your code more expressive — especially if you work in a team. It’s hard to remember all the property values are enabled by default in CSS.