Learn how to join two or more strings with JavaScript.
In JavaScript there are different methods to join two or more strings together, including the plus operator +
, the plus equals +=
operator, and concat()
.
Note: +
and +=
are known as assignment operators.
Let’s try all three!
The plus operator (+)
Let’s say you have two variables, called firstName
and lastName
, and you want assign the combined value of those variables to a third variable, called fullName
. You can do that like this:
const firstName = "Steve"
const lastName = "Jobs"
const fullName = firstName + lastName
console.log(fullName) // SteveJobs
Add separation (space)
To make the fullName
string value more readable, let’s add a space between Steve and Jobs. We’ll reuse same code from before, but this time we add a separator in between + '' +
, like this:
const firstName = "Steve"
const lastName = "Jobs"
const fullName = firstName + " " + lastName
console.log(fullName) // Steve Jobs
Note: you could also add the separator by adding a space after "Steve "
or before " Jobs"
but that can lead to other formatting problems if you’re planning on using the firstName
or lastName
variables in a different context in your project. So I don’t recommend that approach.
The plus equals operator (+=)
If for whatever reason you don’t want to create a new variable (fullName
) you can assign the lastName
variable to firstName
by using the plus equals operator +=
:
console.log((firstName += lastName))
// SteveJobs
However, as you can see, now we need a separator again. To add multiple values with the +=
operator you might think this is possible:
firstName += " " += lastName
But that will throw you an error:
Uncaught SyntaxError: Invalid left-hand side in assignment
Instead you need to combine values on separate lines. First assign the space separator to firstName
, and then assign the lastName
to firstName
afterward:
let firstName = "Steve"
let lastName = "Jobs"
// First assign separator value
firstName += " "
// Then assign last name
firstName += lastName
// Result: Steve Jobs
The concat() method
You can also join strings by using JavaScripts concat()
method:
let firstName = "Steve"
let lastName = "Jobs"
const fullName = firstName.concat(lastName)
console.log(fullName)
// SteveJobs
Once again, we need a separator. That’s straight forward with concat()
. Just add a space with string and a comma, followed by the lastName
variable:
const fullName = firstName.concat(" ", lastName)
console.log(fullName)
// Steve Jobs
Which method should you use to join strings?
For performance reasons, Mozilla and many other credible JS sources strongly recommend using +
or +=
whenever it’s possible.
As this JSPerf test case shows, string objects like concat()
are way slower for the JavaScript interpreter (that runs in your browser) than string primitives like +
and +=
:
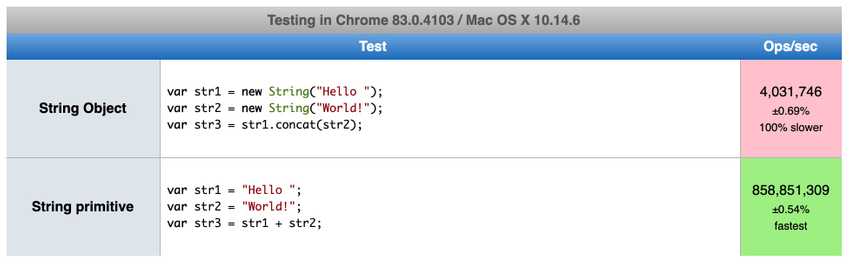