What is an Alert Box?
An Alert Box is a little window that pops up as a response to some type of user interaction (clicking, scrolling, typing, etc.). Alert boxes are mostly used to warn users about issues, but they are also used to merely inform the end-user.
A common example of using alert boxes is if a user clicks the form submit button, but they forgot to fill out a required field. The alert box will then pop up with a message that explains the issue to the user:
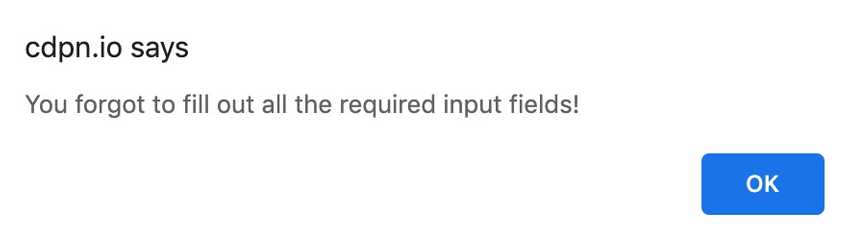
How to create an Alert Box
Let’s replicate the exact alert box from the screenshot above.
First, add create a button element to use as a trigger:
<button onclick="missingFieldWarningPopup()">Submit form</button>
Now add this JavaScript function to your .js
file:
function missingFieldWarningPopup() {
alert("You forgot to fill out all the required input fields!")
}
What happens in the code: the button element has an onclick
attribute as a click event trigger. When the user clicks on the button, the click event calls the missingFieldWarningPopup()
function, which pops up the alert()
prompt window and displays the string (text) inside.
If you want to add the JavaScript function as inline, code, then add this inside the <head>
element of your HTML document:
<script type="text/javascript">
function missingFieldWarningPopup() {
alert("You forgot to fill out all the required input fields!")
}
</script>